A hook to easily select items based on an array
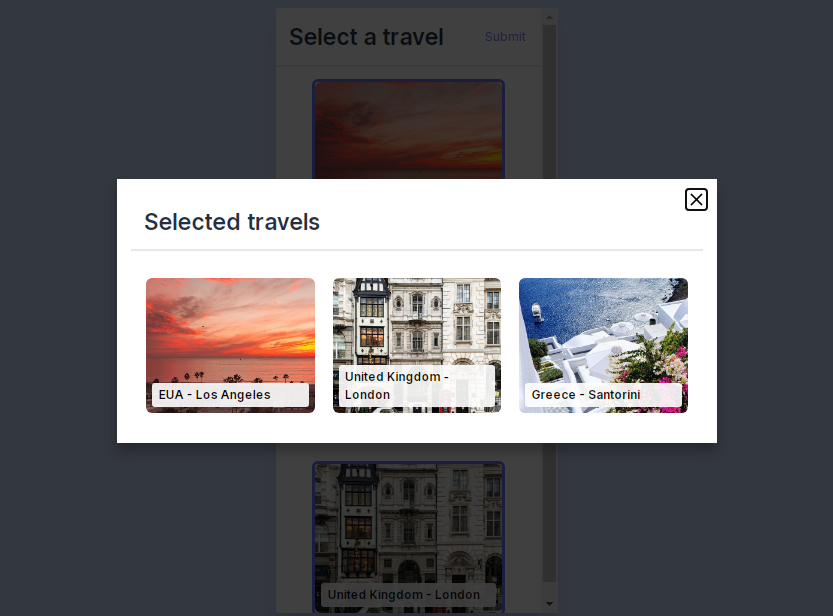
useSelectedItems
Easily select items based on an array.
Installation
With Yarn
yarn add use-selected-items-hook
With NPM
npm install use-selected-items-hook
Usage
import useSelectedItems from "use-selected-items-hook";
const [
selectedItems,
listItems,
{ toggleItem },
] = useSelectedItems<ItemType>({
itemIdentifier: "id",
items,
});
const handleClick = (item) => () => {
toggleItem(item);
};
return (
{
listItems.map((item) => {
// You're able to apply a specify style to a selected item
const itemClasses = classNames("cursor-pointer border-white border-solid", {
"border-black": item.selected,
});
return (
<div
key={item.id}
className={itemClasses}
onClick={handleClick(item)}
>
<p>
{item.name}
</p>
</div>
);
})
}
)
As showed in the example above, you're able to pass an array of items from any type of source, as long it has a unique identifier in
order to compare the items.
API
useSelectedItem
export type Item<T> = T & {
selected: boolean
};
export interface Actions<T> {
toggleItem: (T) => void,
setSelectedItems: Dispatch<SetStateAction<T[]>>,
setItemsList: Dispatch<SetStateAction<Item<T>[]>>
}
useSelectedItems<T>({
itemIdentifier: string | number,
items: T[],
}): [T[], Item<T>[], Actions<T>];
The two arrays returned are the following: selectedItems
and listItems
.
selectedItems
: The items currently selected and that might be send for an API.listItems
: The items with the boolean state ofselected
, which you're able to map and show an visual feedback.
The actions allow you to manipulate items.
toggleItem
: Toggle the selected item, by changing the state and pushing or removing from the array.
Builds
es
(EcmaScript module)cjs
(CommonJS)