Ars Arsenal
A react gallery picker.
Installation
npm install --save ars-arsenal
Styles
Ars Arsenal ships with a stylesheet. The easiest way to include it is
by importing it from the node_modules folder:
/* Sass stylesheet: */
@import "./node_modules/ars-arsenal/style/ars-arsenal.scss"
/* or CSS: */
@import "./node_modules/ars-arsenal/style.css"
Icons
Copy over icons from ./example/public/icons
to your public directory.
Usage
var ArsArsenal = require('ars-arsenal')
var app = document.getElementById('app')
ArsArsenal.render(app, {
resource: 'photo', // the noun used for selection, i.e. "Pick a photo"
url: 'photo/resource/endpoint',
// How to display the items. Can be "table" or "gallery"
mode: 'gallery',
// What table columns to display, and in what order
columns: ['id', 'name', 'caption', 'attribution', 'preview'],
multiselect: false,
makeURL: function (url, id) {
// define how the endpoint url is constructed
if (id) {
return url + "/" + id
}
return url
},
makeQuery: function (term) {
// define how the search query string is built
return "q=" + term
},
onError: function(response) {
// format errors before they are sent as a "string" value
// to the component
return response.code + ": " + response.message
},
onFetch: function (response) {
// format the response, useful if you do not control the JSON
// response from your endpoint
return data
},
onChange: function (id) {
// Whenever a new item is picked, this event is triggered
console.log("The value was changed to %s", id)
}
})
Response format
[
{
"id": 1,
"attribution": "League of Legends",
"name": "Alistar",
"caption": "Lorem ipsum dolor sit amet",
"url": "images/alistar.jpg"
},
//...
]
See example!
Contributing
Setup
npm install -d
npm start
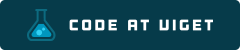