meetME
meetME is a serverless, progressive web application (PWA) with React using a test-driven development (TDD) technique. The application uses the Google Calendar API to fetch upcoming events.
Main view:
After Login, here users can search events from different cities, users can define the number of search results and show or hide a chart which shows the number of events in each citiy.
Modal is open:
If users clicks on 'More details' button a modal is opening and shows more details about the event.
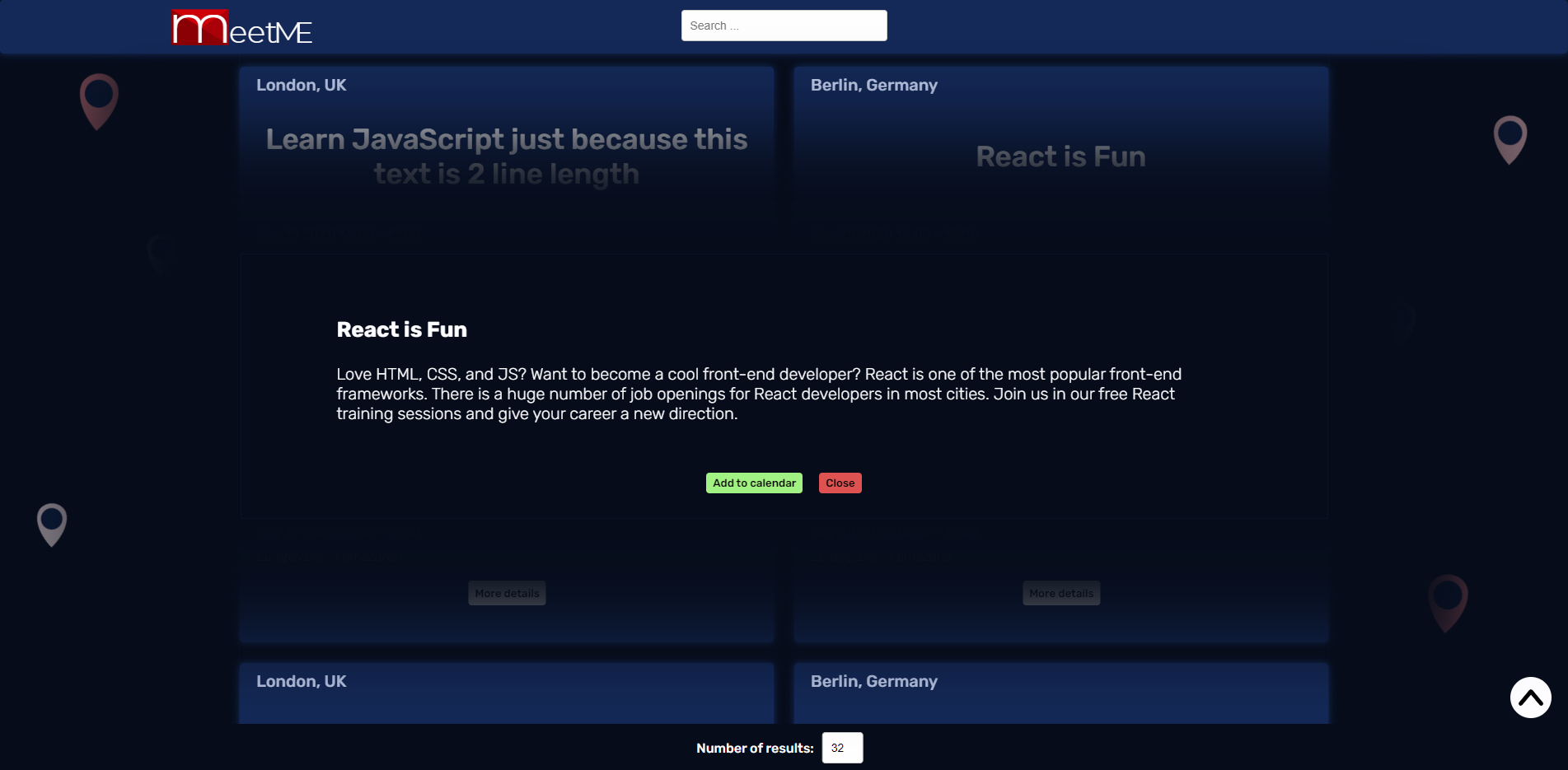
In the project directory, you can run:
npm start
Runs the app in the development mode.
Open http://localhost:3000 to view it in the browser.
The page will reload if you make edits.
You will also see any lint errors in the console.
npm test
Launches the test runner in the interactive watch mode.
npm run build
Builds the app for production to the build folder.
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.
Your app is ready to be deployed!
Features and Requirements
- Filter events by city.
- Show/hide event details.
- Specify number of events.
- Use the app when offline.
- Add an app shortcut to the home screen.
- View a chart showing the number of upcoming events by city
Technical Requirements
- The app must be a React application.
- The app must be built using the TDD technique.
- The app must use the Google Calendar API and OAuth2 authentication flow.
- The app must use serverless functions (AWS lambda is preferred) for the authorization server instead of using a traditional server.
- The app’s code must be hosted in a Git repository on GitHub.
- The app must work on the latest versions of Chrome, Firefox, Safari, Edge, and Opera, as well as on IE11.
- The app must display well on all screen sizes (including mobile and tablet) widths of 1920px and 320px.
- The app must pass Lighthouse’s PWA checklist.
- The app must work offline or in slow network conditions with the help of a service worker.
- Users may be able to install the app on desktop and add the app to their home screen on mobile.
- The app must be deployed on GitHub Pages.
- The API call must use React axios and async/await.
- The app must implement an alert system using an OOP approach to show information to the user.
- The app must make use of data visualization (recharts preferred).
- The app must be covered by tests with a coverage rate >= 90%.
- The app must be monitored using an online monitoring tool.
Dependencies
testing-library/jest-dom - testing-library/react - testing-library/user-event - atatus-spa - axios - moment - nprogress - react - react-dom - react-scripts - recharts - web-vitals - workbox-background-sync - workbox-broadcast-update - workbox-cacheable-response - workbox-core - workbox-expiration - workbox-google-analytics - workbox-navigation-preload - workbox-precaching - workbox-range-requests - workbox-routing - workbox-strategies - workbox-streamsDevDependencies
@wojtekmaj/enzyme-adapter-react-17 - enzyme - jest-cucumber - puppeteer
Project Brief
Gherkin syntax
1. Filter events by city
As a user I should be able to filter events by city So that I can see the list of events that take place in that city
SCENARIO 1:
- When user hasn't searched for a city, show upcoming events from all cities.
Given user hasn’t searched for any city
When the user opens the app
Then the user should see a list of all upcoming events
SCENARIO 2:
- User should see a list of suggestions when they seach for a city.
Given the main page is open
When user starts typing in the city textbox
Then the user should see a list of cities (suggestions) that match what they’ve typed
SCENARIO 3:
- User can select a city from the suggested list.
Given the user was typing “Berlin” in the city textbox
And the list of suggested cities is showing
When the user selects a city (e.g., “Berlin, Germany”) from the list
Then their city should be changed to that city (i.e., “Berlin, Germany”)
And the user should receive a list of upcoming events in that city
2. Show / Hide event details
As a user I should be able to expand event details So that I can view more information about a specific event
SCENARIO 1:
- An event element is collapsed by default
Given that an event is present
When the user selects "view details" or "view more"
Then the element will expand and display the event details
SCENARIO 2:
- User can expand an event to see its details
Given the event list has loaded
When a user selects the event, either link or "view event details"
Then the event page will load, displaying all the event details
SCENARIO 3:
- User can collapse an event to hide its details
Given the user has expanded an events details
When the user selects "collaps" or "close"
Then the expanded element will collapse, hiding the details of the element
3. Specify Number of Events
As a user I should be able to view a specific number of events per page So that I can adjust my page for screen size and load time
SCENARIO 1:
- When user hasn’t specified a number, 32 is the default number
Given the events list has loaded and the user has not specified the number of events to load
When the user opens the event list
Then 32 events should load on the screen (assuming there is at least 32 events)
SCENARIO 2:
- User can change the number of events they want to see
Given the events have loaded
When a user has specified a number (i.e. 5) for the amount of events to view
Then only the specified number (5) of events should load
4. Use the App Offline
As a user I should be able to use the app offline So that I can view event information without having to connect to the internet/use data
SCENARIO 1:
- Show cached data when there’s no internet connection
Given the app has been openned previously with an internet connection
When a user opens the app
Then the previously cached data should be persistent within the app
SCENARIO 2:
- Show error when user changes the settings (city, time range)
Given the app has cached data
When a user changes settings
Then an error should inform them that it requires an internet connection to load new data
5. Data Visualization
As a user I should be able to view the number of events by city So that I can visualize data about where events are taking place
SCENARIO 1:
- Show a chart with the number of upcoming events in each city
Given that there are events loaded
When a user goes to the data page
Then a chart with visualized data showing how many events per city should load.